How to Solve Python Indexerror: List Index Out of Range?
IndexError: ‘list index out of range’ is a Python error that occurs when attempting to access a list item outside the range of the list. In Python, list indexes are used to access or perform actions on list items. For example, you can print them or iterate through them using loops.
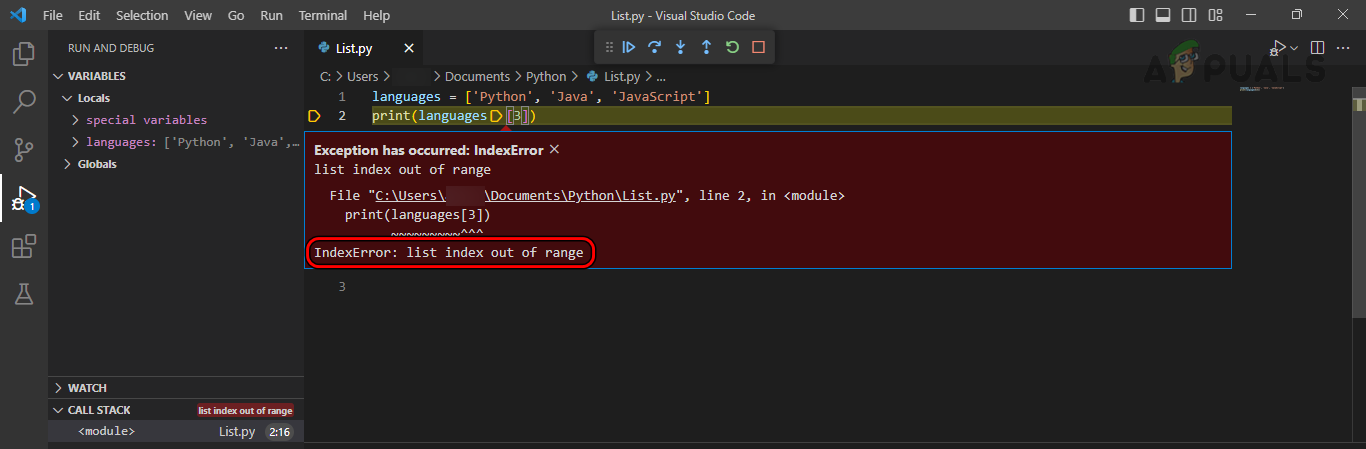
In simple terms, if a list has 5 items and you try to use the 10th item in a list in Python, it will return an IndexError: list index out of range. Usually, these errors are easy to troubleshoot but require a bit of code debugging.
Creating lists in Python.
In Python, a list is created by
- Giving a name to the list
- A space followed by the assignment operator i.e., =.
- A space followed by the opening square bracket.
- Add the first list item in double quotes (not inverted commas).
- A comma and space should follow the second list item in double quotes. Then, you can continue with other list items. At the end of the list, enter the closing square bracket.
For example, to create and print a list of languages in Python:
languages = ["Python", "Java", "JavaScript"] print(languages)
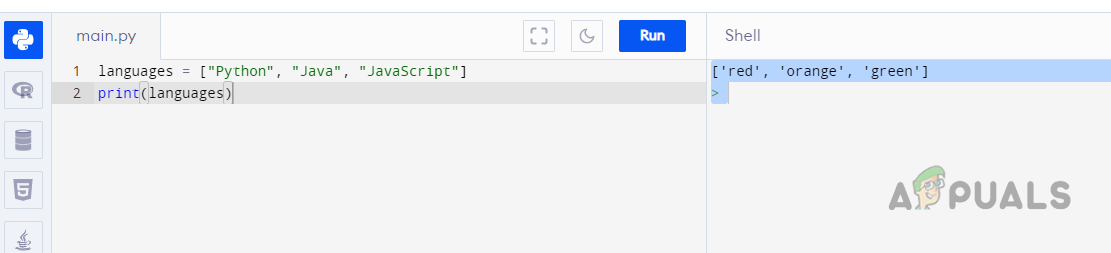
The above code will create a list with the name of languages and contains three entries. First Python, second Java, and third JavaScript.
Check the Length of a Python List
To check the length of the languages list, let us use the length function. To do so, enter the following code:
languages = ["Python", "Java", "JavaScript"] languages_length = len(languages) print(languages_length)
This will return a value of 3.
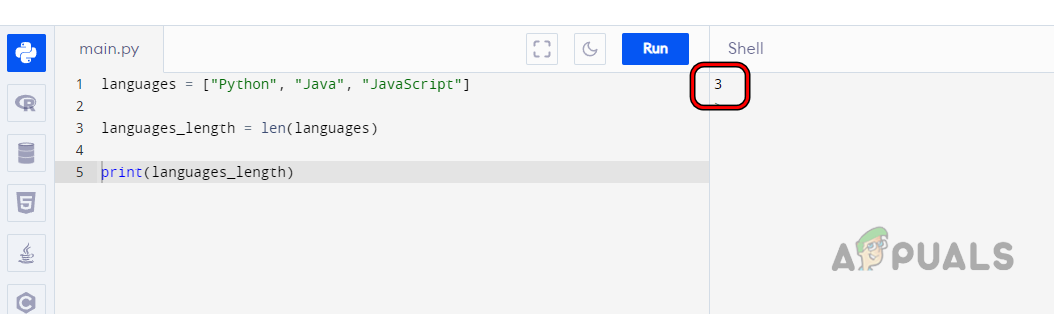
Indexing in Python Lists
In a Python list, each item can be accessed by its index number. Python, like other modern-day languages, is a zero-indexed language i.e., it will start its count from zero (not 1). So, a list with three items will have an index of 0, 1, and 2.
Let us continue with our languages example. To access all its items, use the following code:
languages = ["Python", "Java", "JavaScript"] print(languages[0]) # This will return Python print(languages[1]) # This will return Java print(languages[2]) # # This will return JavaScript
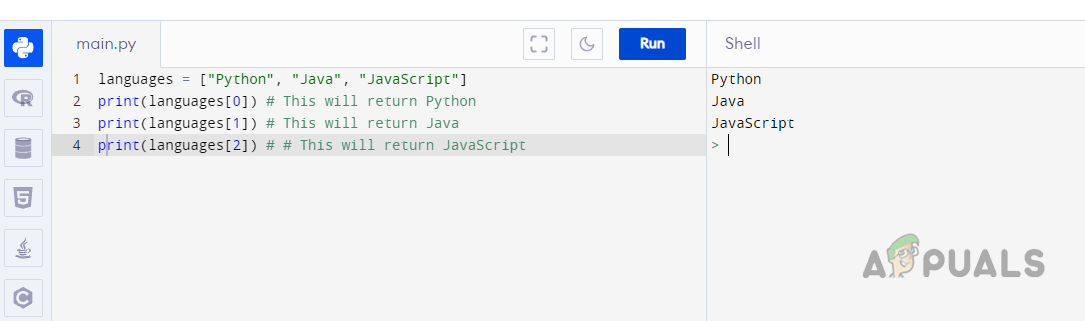
Index Range in a Python List
So, it can be easily said that the index range of a list in Python will be 0 to n-1, where n is the total items in the list. In the above example, the index range will be 0 to 2 (3-1).
Negative Indexing in a Python List
You can also use negative indexing to access a Python list item. The last item will have a -1 index, the second last will have a -2 index, and so on. For example
languages = ["Python", "Java", "JavaScript"] languages[-1] # this will return the last item which is JavaScript languages[-2] # this will return the 2nd last item which is Java languages[-3] # this will return the third last item which is Python
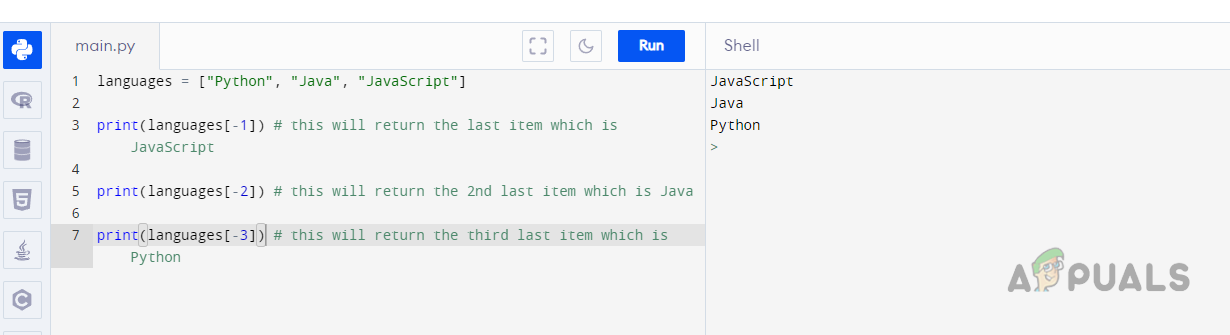
Index Range in Negative Indexing
In the negative indexing of a Python list, the index range will be -1 to -n, where n is the total values present in the list. In our languages list, the negative index range will be -1 to -3 as the length of our list is 3.
Indexerror: List Index Out of Range
Usually, new entrants to the Python world face the Indexerror when, in their code, they start indexing at 1, not zero. Let us clear this by an example. Look at the languages list below:
languages = ['Python', 'Java', 'JavaScript']
It has 3 languages, first Python, second Java, and third JavaScript. Let us try to print the 3rd language in the list:
print(languages[3])
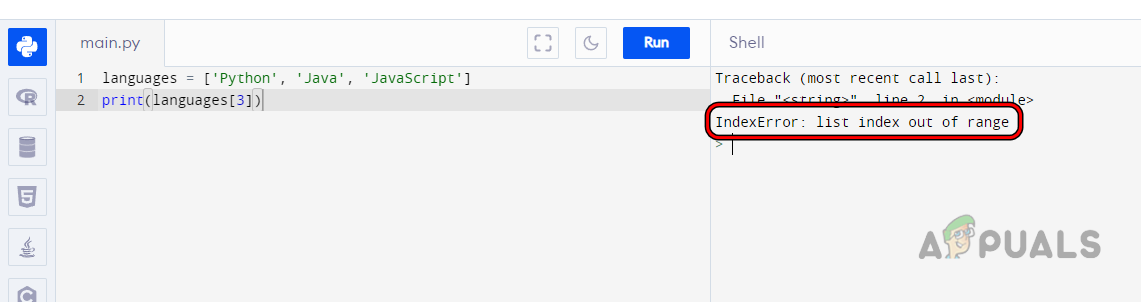
But it will throw the Indexerror. But why? Here, the index range of our list is 3 and we are trying to print the 3rd language in the list. However, in our code, we are asking Python to print the 4th language which is not present and out of the range of the list index. How?
Index 0 = Python Index 1 = Java Index 2 = JavaScript
There is no 4th value so Python throws Indexerror. Here, the proper code will be
languages = ['Python', 'Java', 'JavaScript'] print(languages[2])
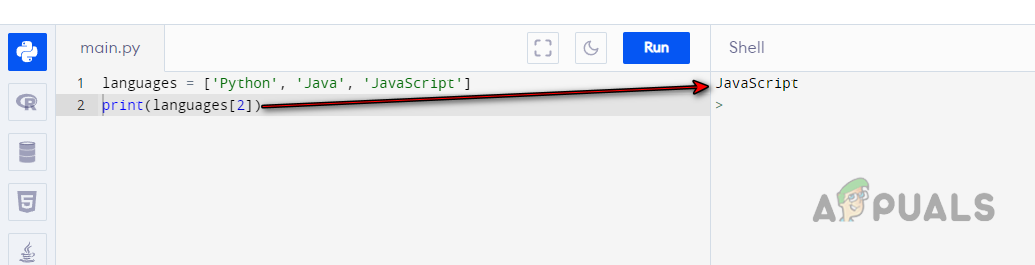
IndexError in Negative Indexing
If we try to print the -4th language in our languages list, we will get the IndexError.
languages = ['Python', 'Java', 'JavaScript'] print(languages[-4])
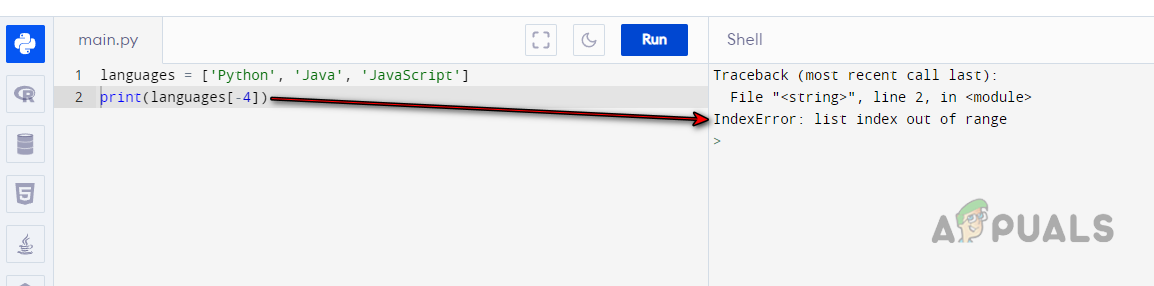
This will throw index error because our index range is -1 to -3, so, there is no -4th value. Here, the proper code would be
languages = ['Python', 'Java', 'JavaScript'] print(languages[-3]) # which will show Python.
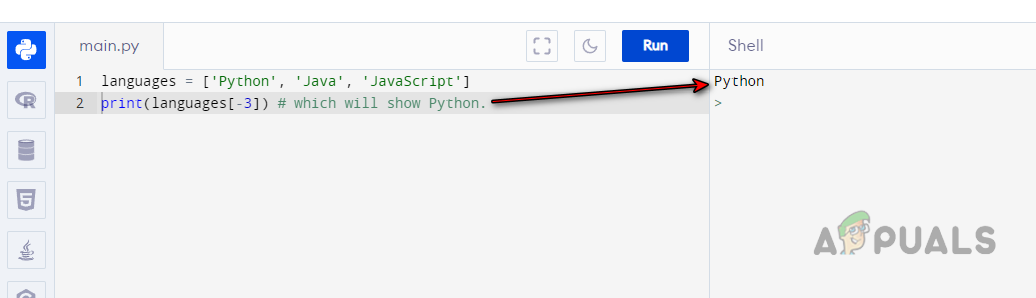
IndexError in Python Loops
Loops in Python, like any other programming language, keep on running until a certain condition is met. A loop in Python will throw an IndexError if the condition specifying the loop involves a list but that condition is invalid as per the list.
IndexError in a While Loop
Look at the code below for a While Loop in Python:
languages = ['Python', 'Java', 'JavaScript'] i = 0 while i <= len(languages): print(languages[i]) i += 1
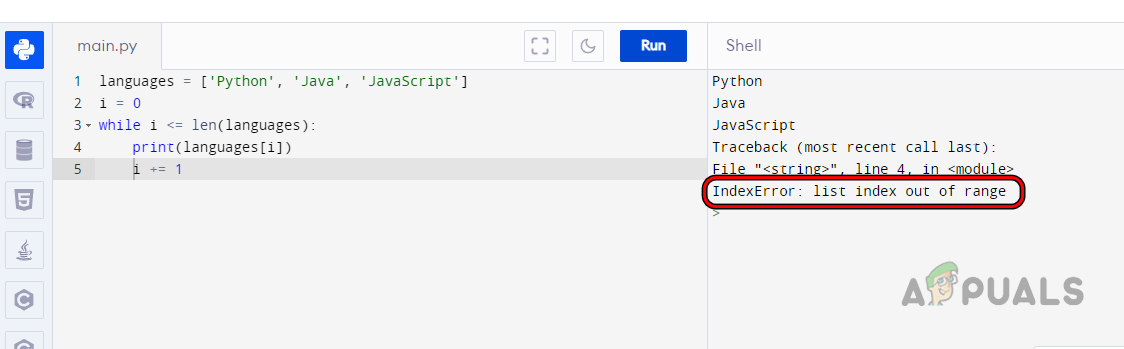
Here, we are asking Python to make a languages list and then declare a variable i with a starting value of 0. Then we announced our while loop and ask the code to be executed till i variable is less than or equal to the length of the list i.e., 3. But this throws an IndexError. Why?
Our list here has an index range of 0 to 2 (3-1).
Index 0 = Python Index 1 = Java Index 2 = JavaScript
But there is nothing in Index 3. But our code is asking the Python compiler to keep on running till the variable i equals 3 which is not possible, hence the out-of-range error.
So, let’s modify our code to run the while loop till the range equals 2, not 3.
languages = ['Python', 'Java', 'JavaScript'] i = 0 while i < len(languages): print(languages[i]) i += 1
So, we removed the equal part and used the condition to run till the variable i is less than 3. This loop will stop after 2 and thus the loop will execute without any issue.
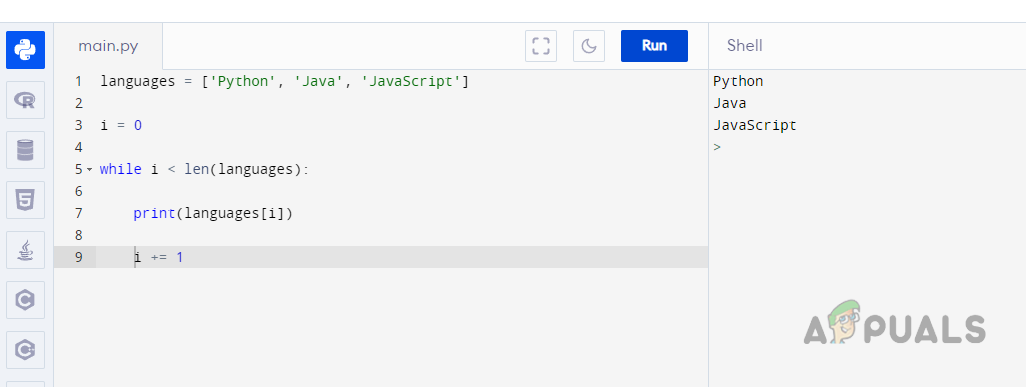
IndexError in the Range Function of a For Loop
The range() function in a Python for loop takes in one integer number for which the counting will stop. The counting in a Range function starts at position 0, then increments by 1 after each iteration, and the number where counting will stop but this number is not included.
The textbook form of a Range() function syntax will be:
range(start, stop, step).
Start: is an optional integer value that indicates the beginning of a series. Its default value is zero if it is left blank.
Stop: is a mandatory integer value that indicates the point at which the execution of the range function should end. This values itself is not included in the execution.
Step: is an optional value that is used to increase the increment after each iteration. If left blank, its default value is one.
But generally, it is used as:
range(stop)
For example, range(4) indicates that the For loop will start from 0 and end when the count reaches 4 i.e., the loop will stop after 3.
Let’s continue with our example of languages, enter the following code:
languages = ["python", "Java", "JavaScript"] for name in range(4): print(languages[name])
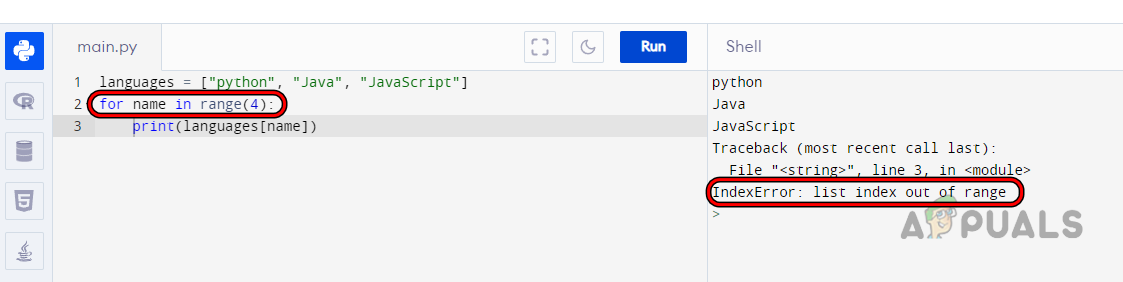
This will again throw an indexerror because the range function will keep on running till the index range of 3 is executed and the count reaches 4. Here, we are asking the interpreter to print the values at index 0, 1, 2, and 3. But the index range is 3.
Index 0 = Python Index 1 = Java Index 2 = JavaScript
So, nothing to execute at Index 3, hence the error. We can correct it by amending our code as:
languages = ["python", "Java", "JavaScript"] for name in range(3): print(languages[name])
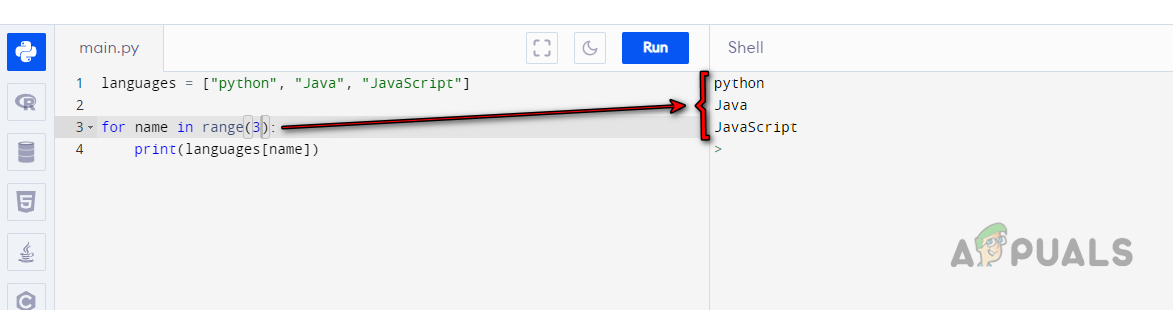
Another way to correct this is by using the length function inside the range function.
languages = ["python", "Java", "JavaScript"] for name in range(len(languages)): print(languages[name])
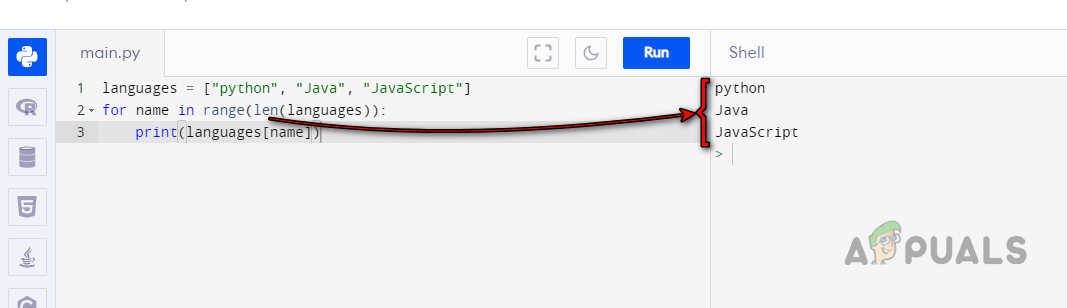
Here, we have instructed the interpreter to stop the count when the length of the languages list (i.e., 3) is reached but not included. So, the interpreter stops after executing the index range of 2 which is a valid entry.
But if you pass a +1 argument to the length function, then it will again cause an indexerror. Look at the code below:
languages = ["python", "Java", "JavaScript"] for name in range(len(languages)=1): print(languages[name])
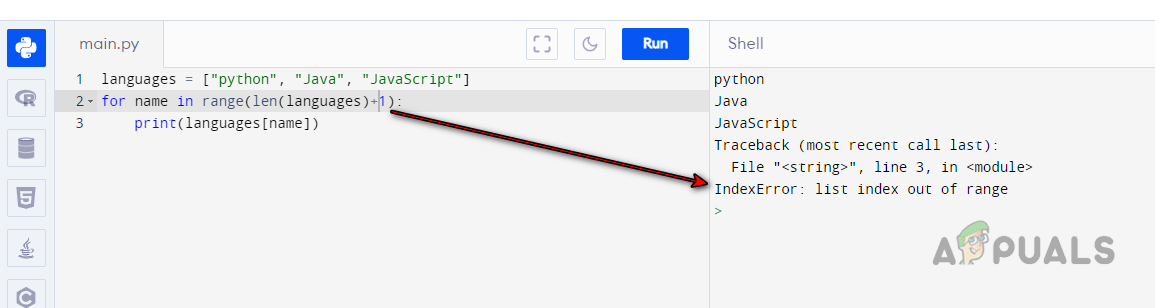
Here, again the count number of the range function reaches four (length of languages list 3+1=4) which is not present, hence the error.
IndexError Due to a Modified List When Iterated
Until now, the list was kept static but if your code removes or adds elements to a list when iterated over it, then that could cause indexerror. Let’s clarify the concept with the following example:
J = [1, 2, 4, 0, 0, 1] for i in range(0, len(J)): if J[i]==0: J.pop(i)
Here, J.pop(i) removes zeros from the list and decreases the list size, and in the next iteration, the index range of the list decreases to 4 but the code is looking for len(J)-1 = 6-1 = 5 which is not present, hence the error.
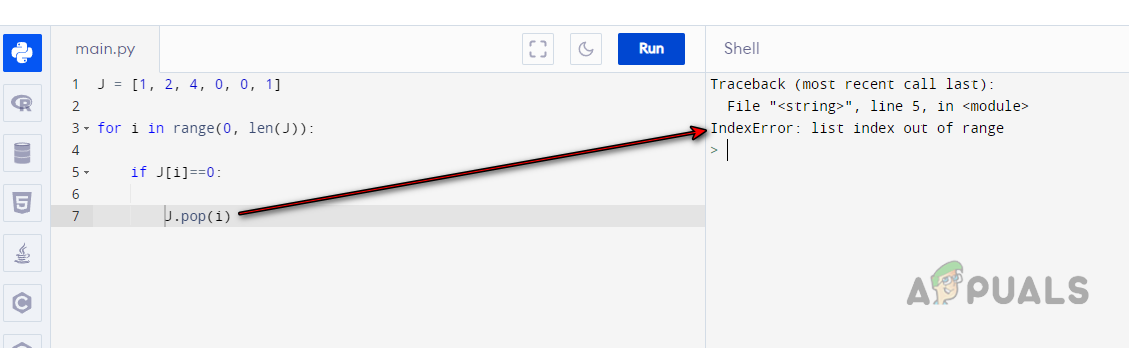
You can fix this by using a list compression and the following code will do the trick.
J = [1,2,3,0,0,1] J = [x for x in J if x != 0] print(J)
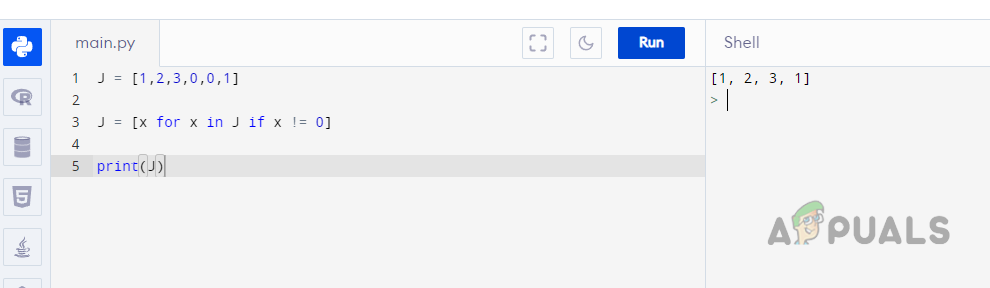
The second list compresses the original list after removing zeros. Another form of this code could be:
J = [1, 2, 4, 0, 0, 1] for i in range(len(J)-1, -1, -1): if J[i] == 0: J.pop(i) print(J)
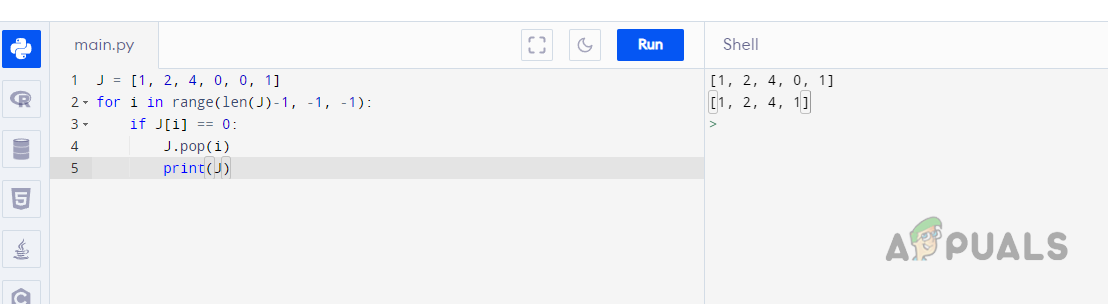
Here is a second example:
colors = ['blue', 'red', 'orange', 'blue', 'green'] for i in range(len(colors)): if colors[i] == 'blue': del colors[i] print(colors)
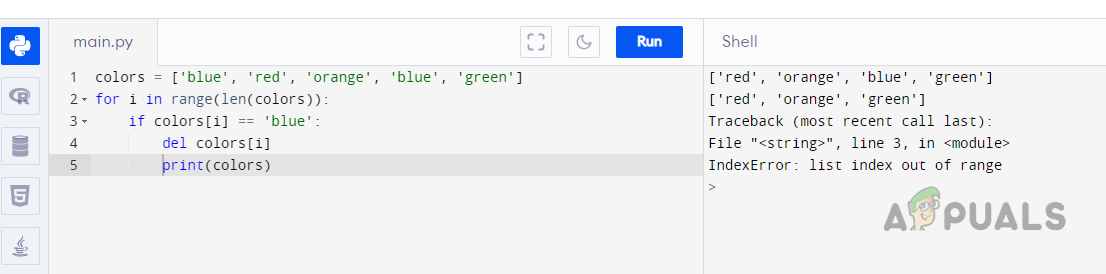
We are trying to remove the blue from the colors list and then print the list but it is throwing an indexerror. This is occurring because the del command is changing the length of the list while in the loop.
At the start of the loop, the length of the colors list is 5, so the range() function generates the index of the list as [0, 1, 2, 3, 4]. With each iteration of the code, the length of the list is decreasing but the index generated by the loop remains 5, and eventually, we will run into an indexerror.
This can be solved by using list compression.
colors = ['blue', 'red', 'orange', 'blue', 'green'] colors = [color for color in colors if color != 'blue'] print(colors)
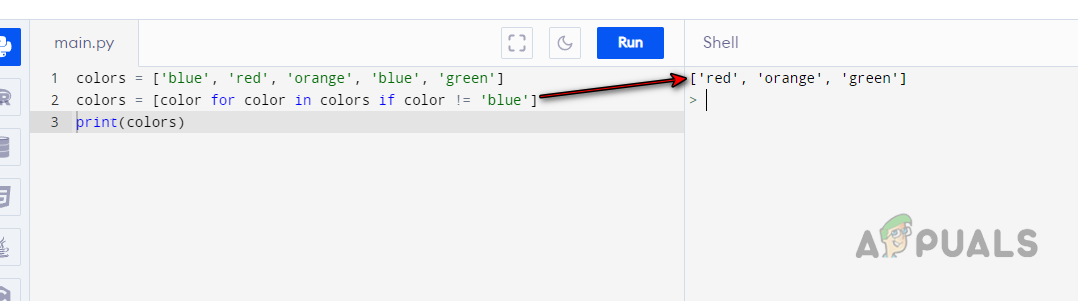
Another form of this code could be:
colors = ['blue', 'red', 'orange', 'blue', 'green'] for i in range(len(colors)-1, -1, -1): if colors[i] == 'blue': del colors[i] print(colors)
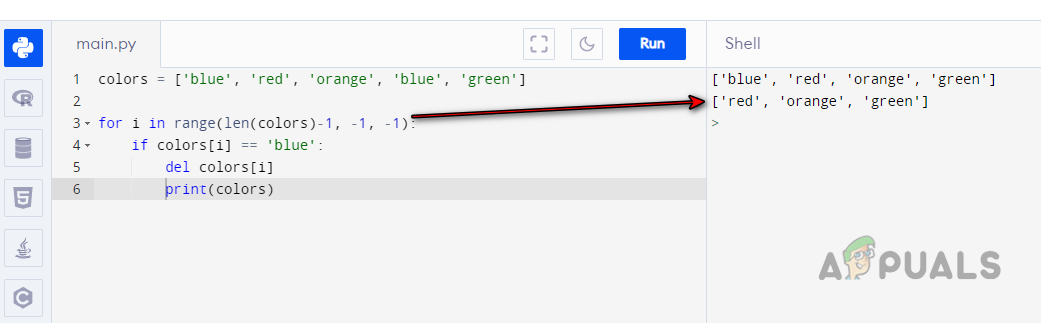
Empty Lists
There can be cases where a list might be empty or got empty after each iteration of the code. When these empty lists are called in your code, these will cause indexerror: List Index Out of Range. Look at the code below:
languages = [] print(languages[0])
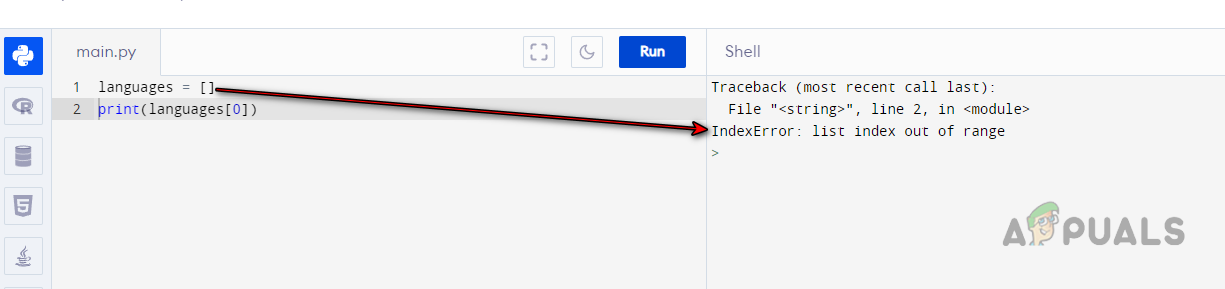
This will throw an indexerror as the list is empty and there is no value at index 0. You can cover this by using IF. The code will be as:
languages = [] if languages == []: print('Empty list') else: print(languages[0])
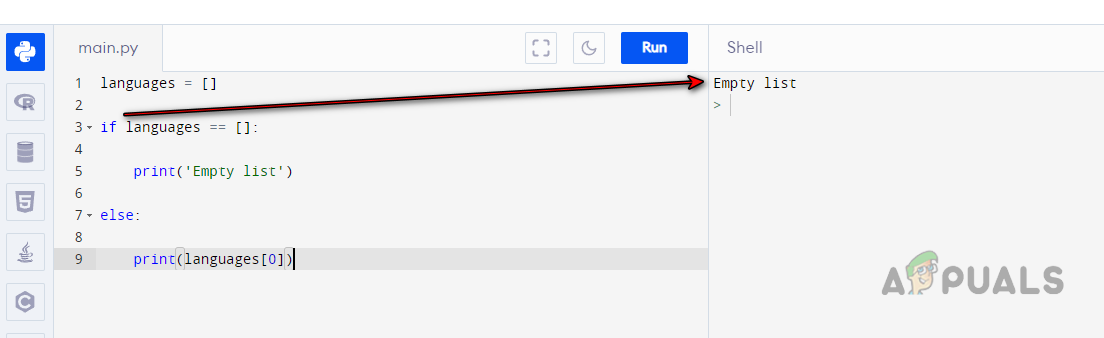
IndexError in a Python String
This error can also occur with Python strings. Let us get it cleared by using an example.
s = 'Python' print(s[6])
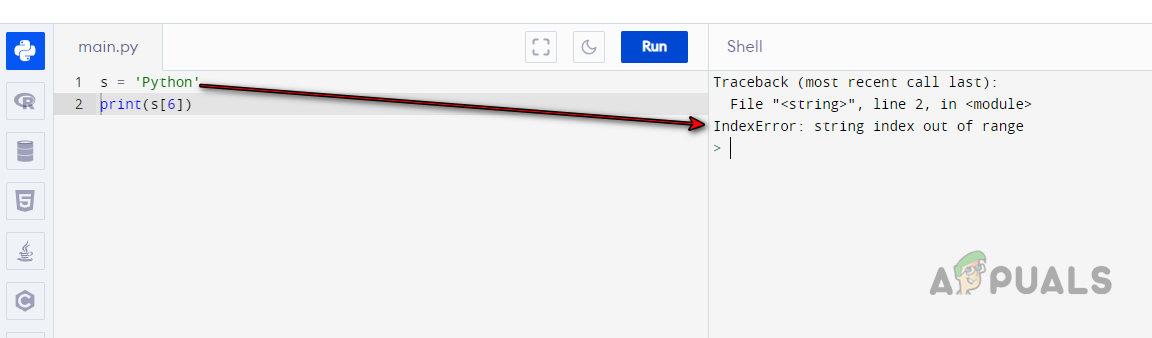
This will throw an indexerror. In this example, we are trying to print the 6th character in the string but instructing the interpreter to print the 7th character which is not present. How? Our 6th character will be indexed as 5 (count from 0 to 5). So, the code should be
s = 'Python' print(s[5])
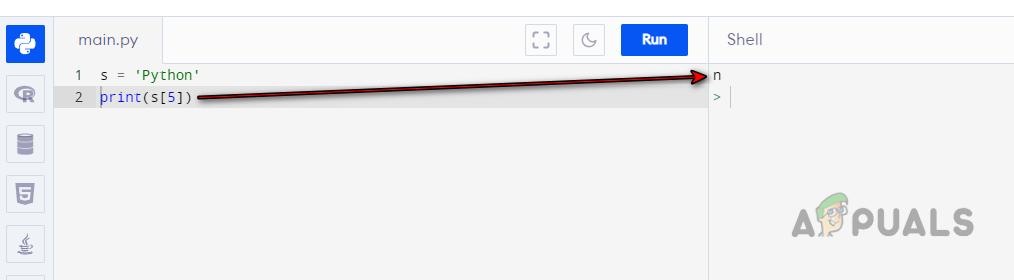
IndexError in a Python Tuple
An IndexError can also occur in a Python tuple. Let us look at the following example:
s = ('Asia', 'Europe') print(s[2])

This will throw an indexerror as we want to call a second entry but eventually call a non-present 3rd entry. The correct code would be:
s = ('Asia', 'Europe') print(s[1])
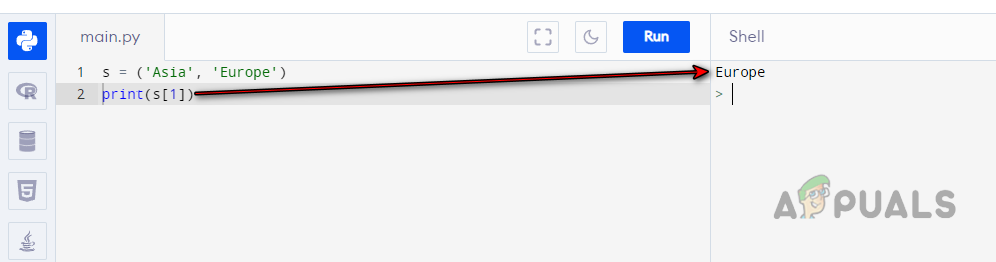
Check for a Library or IDE Bug
If your code is 100% correct but you are still facing an indexerror, then the issue could be a bug in a Python library or IDE. In the recent past, Manim has been reported to throw an indexerror even with its test examples. In such a case, either check your code on another machine or use an online Python compiler for testing.
Causes of IndexError: List Index Out of Range
So, in a nutshell, we can say that an Indexerror: List Index out of Range mainly occurs when:
- You try to access an index that is not present in a list.
- You are using invalid indexes in your loops.
- When you specify a range that is beyond the index range of a list when using the range() function.
- A library or IDE bug.
Method to Solve an IndexError
We have discussed the matter in quite detail but these are all sample codes and you have to dig your code to clear the error. The best method is to note down the line where you are facing the error and just before that, add a print command. This way you will easily be able to note down the issue.
So, readers if you are still confused about the IndexError, you are more than welcome in the comments section.